Question: Tell me the difference between an iterator and an enumeration interface?
Did that shock you? You read that topic but you hadn’t refreshed it the previous day and missed a question you should have answered in the interview. If this sounds familiar then stop blaming yourself and read on.
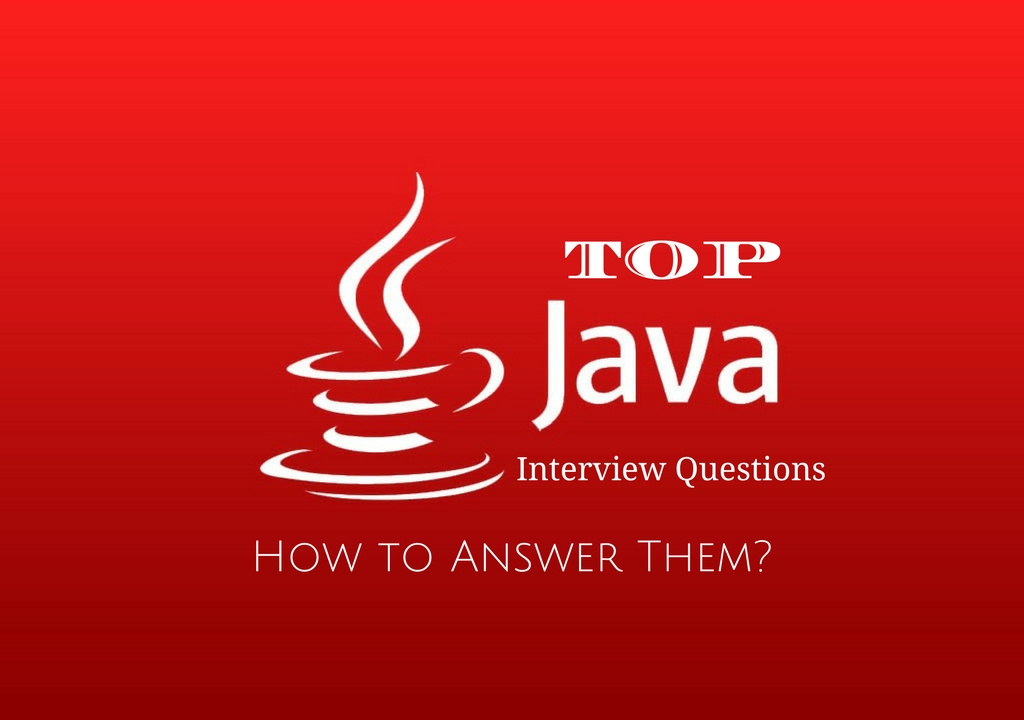
Interviewing for JAVA can be very hard if you aren’t prepared and the subject is so vast, it’s not always possible to know everything all the time. Companies usually hire individuals who have a better understanding of the subject compared to other candidates and usually higher than their benchmark.
Interview question can be asked from anywhere and it’s tough to read and keep in mind everything the night before. Choosing some important questions may help in the way. In this article, we will be listing some of the most asked and important questions on JAVA.
Before we get to the questions there is an important task of building out a proper resume.
Resume Building:
The first thing is to build a resume, that is good enough in JAVA and gets you shortlisted. So mention all the relevant training or certifications programs you have done. Then mention JAVA as a language of interest and put all the projects you have done in the projects section of the resume.
Mention and describe the details of all the project what it does, how it was made, which technologies were used and why it is better than the existing system. Mention any advanced levels that you might have completed or worked on.
For Developers who want to build a career in Java the following list will give you some of the most important questions which are mostly asked at the time of interview.
Java Interview Questions with Answers:
1. What is an immutable object? Give an example.
The Java classes whose objects cannot be modified once created are called immutable classes. If you modify the immutable object then it will create a new object.
Example:
String is an immutable object. Basically immutable objects are final in nature for preventing method overriding.
2. State the difference between JDK and JRE?
This is the most common question in Java interviews as many people confuse these terms. JDK is Java Development Kit and it is a bundle of software that is used to develop Java based Software. It contains JRE, Java source compilers, deployment tools and all the development tools in it.
JRE -Java Runtime Environment is an implementation of the Java Virtual Machine that executes Java programs. It contains all set of libraries plus all other files that JVM uses at runtime.
3. What is the difference between String Buffer and String Builder?
String builder methods are synchronized which means two threads cannot call the methods at the same time. String Buffers are not synchronized that means it is thread safe. String builder is also more efficient than String Buffer.
4. What do you mean by Exception Handling?
Exceptions are objects in Java. When an exception is thrown basically we throw an object. Throwable is the base class for the entire class family that is declared in java.lang. It is the process of handling errors so that the program can execute successfully without any errors. It helps in maintaining the normal flow of the execution. There are two types of Exceptions – Checked Exception and Unchecked Exception.
5. What is the difference between Unchecked Exception, Checked Exception and Error?
Unchecked Exception:
The class which extends Runtime Exception is called Unchecked Exception.
Example:
ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. They are basically checked at runtime.
Checked Exception:
The class which extends Throwable class except RuntimeException and Error is known as checked Exception.
Example:
IOException, SQLException etc. It is checked at compiled time rather than the previous one.
Errors – It is thrown for more complex problems for example OutofMemoryError.
6. What is Singleton Class?
It is a java class with only one instance in whole java application.
Example:
java.lang.Runtime. It can be easily created by Enum. Singleton class controls the access to all the resources like database connection or sockets.
7. What is the difference between Throw and Throws keyword?
Throw:
It is used to explicitly throw an exception. You cannot propagate checked option using throw. It is followed by an instance and used within a method. Throwing multiple exceptions is not possible.
Example:
void a(){
throw new ArithmeticException(“hello”);
}
Throws:
This keyword is used to declare an exception. You can propagate checked exception by and it is followed by a class and used within the class signature. One can easily declare multiple exceptions like public void method()throws IOException, SQLException.
Example:
void a()throws ArithmeticException{
//method code
}
Java throw and throws example:
void a()throws ArithmeticException{
throw new ArithmeticException(“hello”);
}
8. What is FileReader and FileWriter? Write code for each.
Java FileWriter class is used for writing character-oriented data. It is also used for file handling. With the help of this class, you don’t have to convert a string into a byte array as it provides methods so that you can write string directly.
Example:
import java.io.FileWriter;
public class File {
public static void main(String args[]){
try{
FileWriter fwr=new FileWriter(“E:\\test.txt”);
fwr.write(“Hello.”);
fwr.close();
}catch(Exception ex){System.out.println(ex);}
System.out.println(“Yaay…”);
}
}
Assume it is written “Hello” in the “test.txt” file:
Output: Yaay
Java FileReader helps to read data from any external file. Like fileInputStream it returns data in byte format. It is basically used for file handling in Java as it is a character oriented class.
Example:
import java.io.FileReader;
public class FileReader {
public static void main(String args[])throws Exception{
FileReader fre=new FileReader(“E:\\test.txt”);
int a;
while((a=fre.read())!=-1)
System.out.print((char)a);
fre.close();
}
}
Let’s say it is written “Hello” in the “test.txt” file:
Output: Hello
9. What is the basic difference between ArrayList and LinkedList?
This is one of the most important questions asked in Java interviews. ArrayList uses a dynamic array to store elements whereas LinkedList uses the double linked list to store. Manipulation or changing things is a bit slow in ArrayList than the other one. LinkedList can act as a list and also as a queue but ArrayList acts only as a list. So for storing and accessing ArrayList is better and for manipulation and changes, LinkedList is better.
10. Sleep() vs Wait()
Sleep() is used to hold the processes for few seconds but in the case of wait() the thread goes into the waiting state and it won’t come back automatically until you call notify() or notifyAll().
Another major difference is that wait() releases the lock but sleep() doesn’t. The wait is basically used for inter-thread communication but sleep is used to pause on execution.
11. Can we overload static methods?
Yes, it is possible to overload static methods. We need to have multiple static methods with same name but different parameters.
Example:
public class StaticTest {
public static void big() {
System.out.println(“StaticTest.big() called “);
}
public static void big(int a) {
System.out.println(“StaticTest.big(int) called “);
}
public static void main(String args[])
{
StaticTest.big();
StaticTest.big(50);
}
}
Output: StaticTest.big() called
StaticTest.big(int) called
12. What is Classloader? Explain each one of them.
Classloader is a part of the Java Runtime Environment which loads the classes into the Java Virtual Machine dynamically or on demand. It can load the classes from the local system, from the web and even from any remote system. There are three classloaders –
1. Bootstrap Classloader- It loads core Java API
2. Extension Classloader- It loads all the jar files from the folders
3. System/ Application Classloader- It loads jar files from the specified path in the classpath environment variable.
13. What is the difference between an Inner Class and a Sub-Class?
A class which is nested within another class is called Inner Class. It has access to the class which is nesting it and it can also have the permission to access all the variables and methods defined in the outer class.
The class which inherits from another class is called Sub Class. It can also access all public and protected methods and fields defined in the super class.
14. Explain Final Keyword in Java?
When you want to make something constant you can use Final for that. After declaring something final, the value can be assigned once only and after that you can’t change the value. When a method is declared final it cannot be overridden by any subclasses. It is resolved by the compiler at the time of compilation. When you declare a class final then the class cannot be subclassed.
15. What’s the difference between an Abstract Class and Interface in Java?
The main difference between abstract class and interface is that interface can only have abstract methods whereas abstract classes will have both abstract and non-abstract methods. An abstract class doesn’t support multiple inheritances and it can have final, non-final, static and non-static variables. But with the interface you can do multiple inheritances in Java and it only has static and final variables. The classes in Java can implement interfaces but it can only extend the abstract classes.
16. Describe all the parts of the main function in JAVA.
Write the main function if possible in any white page. Then start explaining marking one after another.
- public static void main(String[] args)
- public – means that the method is accessible everywhere
- static means you don’t have to create an instance of the class to call the method.
- void means the main method returns nothing.
- main is the predefined method name.
- String[] means the main method accepts a string of arrays as parameters.
- args is the parameter name. You can name it anything you want.
17. What is a Super keyword? Give an example.
It is a reference variable which is used to refer to the immediate parent class object. When we create an instance of a subclass, an instance of the parent class is implicitly created and referred by the super reference variable.
Use:
- It is used to refer to the immediate parent class instance variable.
- Super can also be used to call the immediate parent class method.
- Super() can also be used to invoke immediate parent class constructor.
18. State the difference between final, finally and finalize.
Final:
It is a keyword which is used for restriction on classes, methods, and variables. You can’t inherit final classes or override final methods and change the values of the final variable.
Example:
class FinalExam{
public static void main(String[] args){
final int x=100;
x=200 //Compile Time Error
}}
Finally:
It is a block which is used to place some code which no matters what you need to execute even if any exception is handled or not.
Finally example:
class FinallyExam{
public static void main(String[] args){
try{
int x=300;
}catch(Exception e){System.out.println(e);}
finally{System.out.println(“finally block is executed”);}
}}
Finalize:
It is a method which is used to perform garbage cleaning of objects in JAVA.
Finalize example:
class FinalizeExam{
public void finalize(){System.out.println(“finalize is being called”);}
public static void main(String[] args){
FinalizeExam f1=new FinalizeExam ();
FinalizeExam f2=new FinalizeExam ();
f1=null;
f2=null;
System.gc();
}}
19. Difference between method Overloading and Overriding.
Method Overloading increases the readability of the program. If a class has multiple methods and all the methods name is same then you can overload them with different parameters to work differently. You can overload by changing the return types or changing the number of parameters also.
Example:
class Add{
static int addmethod(int a,int b){return a*b;}
static int addmethod(int a,int b,int c){return a+b+c;}
}
class Overloading1{
public static void main(String[] args){
System.out.println(Add.addmethod(10,2));
System.out.println(Add.addmethod(10,11,12));
}}
Output: 20
33
Method Overriding:
If the subclass or the child class has the same method as declared in the parent class then it is known as Method overriding.
Example:
class Car{
void fly(){System.out.println(“Car is running”);}
}
class Bike extends Car{
void fly(){System.out.println(“Bike is running “);}
public static void main(String args[]){
Bike obj = new Bike();
obj.fly();
}
}
Output: Bike is running.
20. How to avoid deadlock?
By breaking the circular wait condition we can get out of the deadlock.
- Don’t use multiple deadlocks
- Don’t use multiple locks at once and don’t execute foreign code while holding a lock
- Always acquire the locks in the same order
While this list of questions is not exhaustive it does give you the key areas, the interviewers want to test you on. If you are starting out learning Java and interviewing with Jobs the Interviewer will ask you questions which test your understanding on Fundamental concepts of Java.
We suggest that you read, practice and code a few examples on some of these topics to get more detailed understanding of these concepts.
Walk into your next interview with more confidence and read as much as you can and practice.